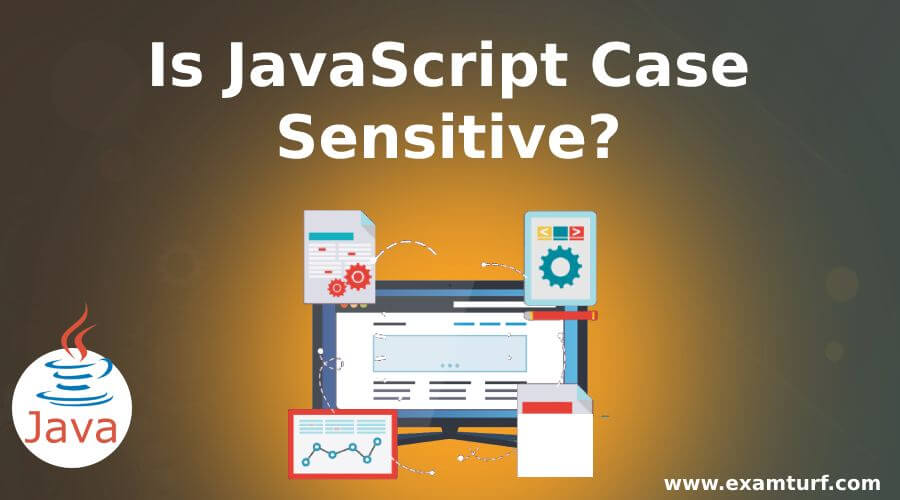
Introduction to JavaScript
JavaScript is an object-oriented, lightweight, prototype-based interpreted scripting language for web pages. Similar to other programming or scripting languages, JavaScript also has a set of rules while writing the code and one of such rules is Case sensitivity. Case sensitivity refers to that keywords, variables, function names, and some identifiers need to have the same capitalization of words. For example, the keyword “while” needs to be typed with the same spelling and the same word capitals. It can’t be typed as “While” or “WHILE,” where words are the same but capitalization is different. This condition is called Case sensitive, and JavaScript is Case sensitive. Now we know that in JavaScript, functions and keywords need to be case-sensitive.
Table of contents
Guidance to Is JavaScript Case Sensitive?
Given below shows guidance to Is JavaScript Case Sensitive:
1. Keywords in JavaScript
These are reserved words that cannot be used as variables or function names.
These are just syntax in JavaScript which will be used to write code.
Syntax:
const x = ‘hey world’
Here “const” refers to keyword, and “x” is a variable, and const cannot be used for anything else other than the syntax for code.
Given below are some of the keywords for JavaScript:
abstract | default | function | null | throws |
arguments | delete | goto | package | transient |
await | do | if | private | true |
boolean | double | implements | protected | try |
break | else | in | public | typeof |
byte | enum | instance of | return | var |
case | eval | int | short | void |
catch | false | interface | static | volatile |
char | final | let | switch | while |
const | finally | long | synchronized | with |
continue | float | native | this | yield |
debugger | for | new | throw |
2. Variables in JavaScript
Variables are used for storing information as they act as a vessel. The variable can be declared using the keyword “var” and is case-sensitive.
Example:
Code:
var a = 10;
var b = 5;
var c = a b
- The variable ‘a’ will be stored as 10.
- The variable ‘b’ will store as 5.
- The variable ‘c’ will store as 15.
The JavaScript version ES6-ECMAScript 2015 will have the privilege to use “const” and “let”. For const, variables used once cannot be reassigned. For let, the variables are defined with restricted scope.
3. Functions in JavaScript
- Function Definition: These are used for performing a particular task. Functions take users’ input, do some computation, and then generate output. Commonly and repeated calculations or tasks can be performed using functions, and writing the same code repeatedly can be avoided, and it saves a lot of time for programmers.
- Function Syntax: To create a function, we need to use a function keyword, followed by a function name, followed by parameters which are placed inside common brackets(). Function names can be letters, digits, or dollar signs (same rules as variables). The code to be executed by function is placed inside flower brackets {}.
Syntax:
function functionName(parameter1, parameter2, ….){
//code to be executed
}
Example:
Code:
function sumNumber (number1, number2)
{
return number1 number2;
}
In the above example, we have created a function named sum number. This function receives 2 numbers as parameters and gives the output as the sum of these 2 numbers.
4. Invoking a Function
After writing a function, the next step is to utilize the function. We can invoke a function by using the function name parted by the parameters enclosed between common brackets and a semicolon at the end.
Syntax:
functionName(parameter1, parameter2, ..);
Example:
Code:
<script type = "text/javascript">
// Function definition
function welcomeMsg(name) {
document.write("Hey" name " welcome to EXAMTURF");
}
// creating a variable
var nameVal = "Admin";
// calling the function
welcomeMsg(nameVal);
</script>
Output:

- Return Statement: These are used to return the function after the task is completed. This is an optional statement; most of the time, it’s the last statement in a JavaScript function. In the above example of a sum number, the function calculates the sum of 2 numbers and then returns the result.
syntax
return value;
- Local Variables: Variables assigned within a JavaScript function become local to the function. Local variables are only identified inside their functions. Thus, variables with the same name can be used in different functions. Local variables are created when a function is called and canceled when the function is completed.
Example:
Code:
// code here can NOT use watchName
function myFunction() {
var carName = "Sonata";
// code here CAN use watchName
}
// code here can NOT use watchName
Recommended Articles
This is a guide to Is JavaScript Case Sensitive? Here we discuss the introduction and guidance to JavaScript case sensitive. You can also go through our other suggested articles to learn more –
Are you preparing for the entrance exam ?
Join our Programming Languages test series to get more practice in your preparation
View More